Let’s talk Salesforce collection variables, those handy flow resources that let you store items in a list (eg, records, numbers, text, etc.). Although it’s more likely you’ll simply want to add items to your collection, occasionally you may find that you actually need to empty one (clear it out) so it can be used again.
To do this in Apex, we would simply use the clear() method to remove all the items at once (see line 3 below):
List<String> myColorList = new List<String>{'red', 'green', 'blue'}; System.debug('myColorList: ' + myColorList); myColorList.clear(); System.debug('myColorList: ' + myColorList);
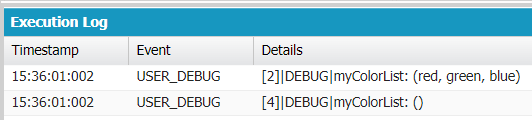
In Flow Builder, there are two ways to empty a collection.
The first is pretty straightforward: Use an Assignment Element and simply make the collection equal to nothing (just keep the value blank).
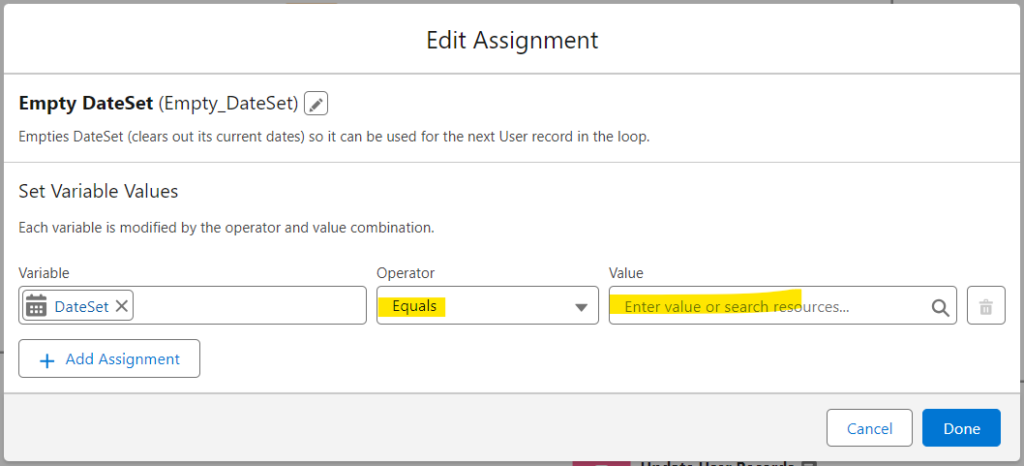
For the second method, we need to use the Remove All operator — which does exactly what you think it would do but requires some explanation to use correctly.
The trick with Remove All is that we have to present it with a collection of items (the reference collection) that we want removed from our original collection (the target collection). In doing this, the flow will first look at the items in the reference collection on the right and then remove them from the target collection on the left.
Fortunately, both the target and reference collections can be the same collection — and this is how we empty out our collection variable.
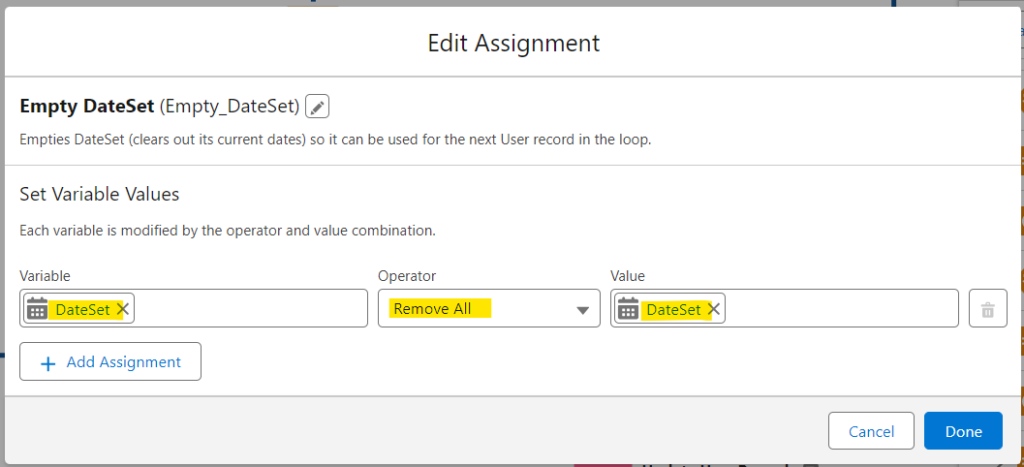
As you can see above, both the variable (target collection) and value (reference collection) are the same. This ensures everything is emptied out of our target collection.
An important point you need to know is that once you empty your collection variable (regardless of the method), it will NOT equal null. Instead, it will simply be empty. (Not surprisingly, this is also true in Apex as we saw in the code above.)
For this reason, any null check on the newly emptied collection will no longer result in true. The same is true for simply checking for empty (no value).
This can be confusing since a newly created collection variable in your flow is null and would return true for a null check. But once filled and emptied, it’s no longer null. So, at this point, the only way to check for empty is to check the size of the collection.
You do this by creating a new number resource variable and using the Equals Count operator on the collection variable you want the size of.
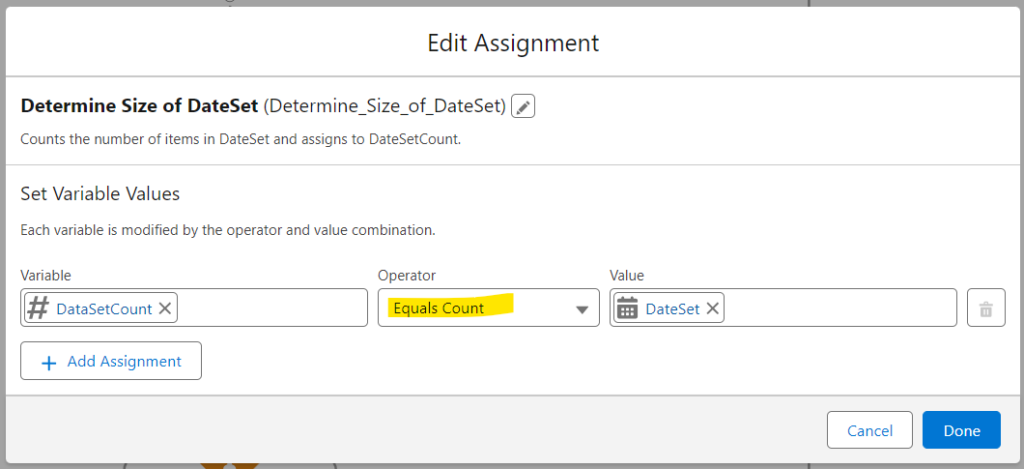
Then you simply need to check the size (count) of the collection variable in a Decision Element. If it equals zero, then the collection variable is empty.
Now, to bring it full circle back to Apex, here’s additional code that checks for null, empty, and size (see lines 5-7):
List<String> myColorList = new List<String>{'red', 'green', 'blue'}; System.debug('myColorList: ' + myColorList); myColorList.clear(); System.debug('myColorList: ' + myColorList); System.debug('myColorList is null: ' + (myColorList == null)); System.debug('myColorList is empty: ' + myColorList.isEmpty()); System.debug('myColorList size is: ' + myColorList.size());
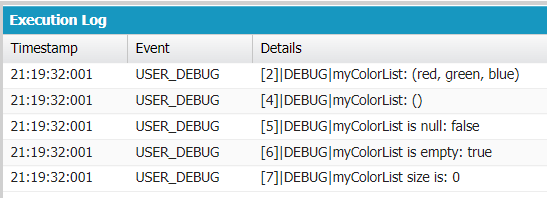
Can you think of another way to clear out a collection variable in a Salesforce flow? Did I get something crazy wrong above? If so, just let me know in the comments below!
Thank you for the article, being a new Apex learner I like how you compare Flow and Apex. I’m inspired to do that now.
Just one little thing I just tested: in Apex if you create a new list, it’s considered empty, not null. Looks like Flow and Apex don’t agree on this matter? 😀
Thank you for the comment. So, the distinction really is between declaring a variable and initializing it.
If you simply declare a list in Apex:
List<Account> accts;
it will be null. But if you initialize the list:List<Account> accts = new List<Account>();
it will be empty.For this reason, I’m under the impression that if you create a resource in Flow Builder but don’t give it a default value, then it’s simply being declared and not initialized. This would make it null. (As you can imagine, Salesforce is very resource-protective and avoids expending resources (eg, memory) for something that may not even be used.)
I imagine Flow Builder later initializes that variable once you try to add (or assign) a value to it. If that sounds like faulty logic, let me know!
Ah I see, I forgot the difference between declaring and initializing a variable. Thanks for clarifying that.
Sure — thanks for asking the question!